Lifecycle and States of a Thread in Java (to be continued)
This article is taking this great article as a reference.
A thread in Java has the follownig possible states:
NEW, RUNNABLE, TIMEDWAITING, WAITING, BLOCKED, TERMINATED
The diagrams shown below represent the relationships between different states of a thread at any instant of time.
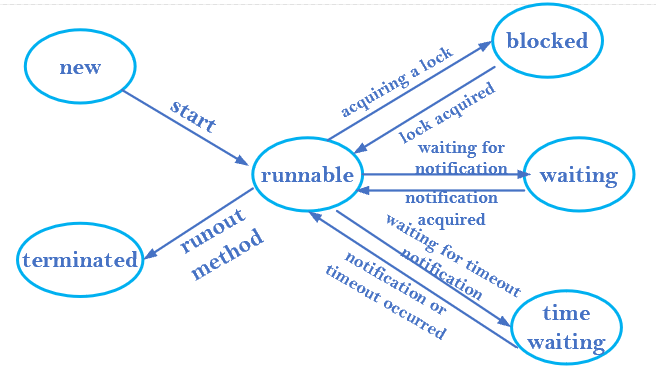
Image Source: Lifecycle and States of a Thread in Java - geeksforgeeks
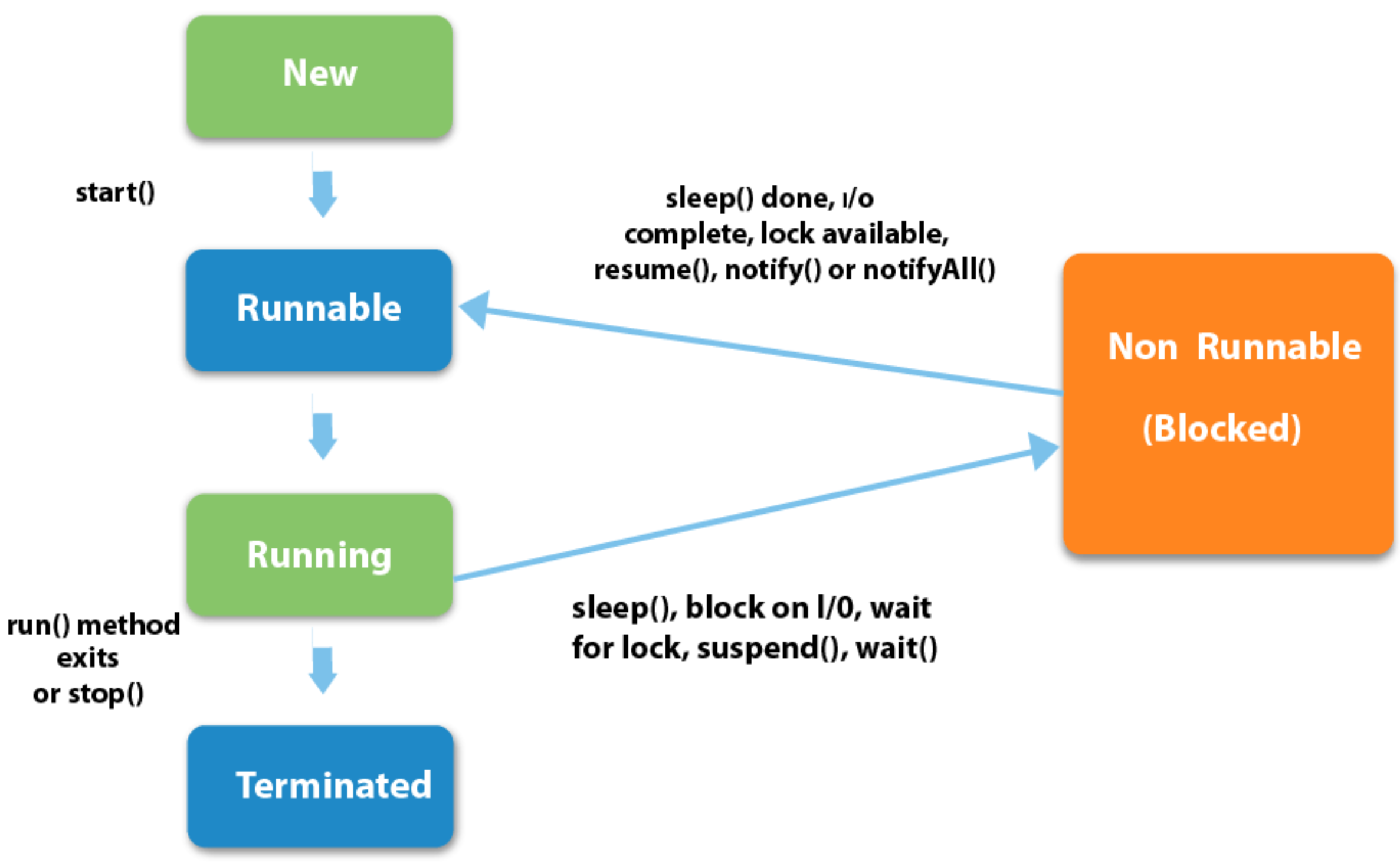
Image Source: Life cycle of a Thread (Thread States) - java T point
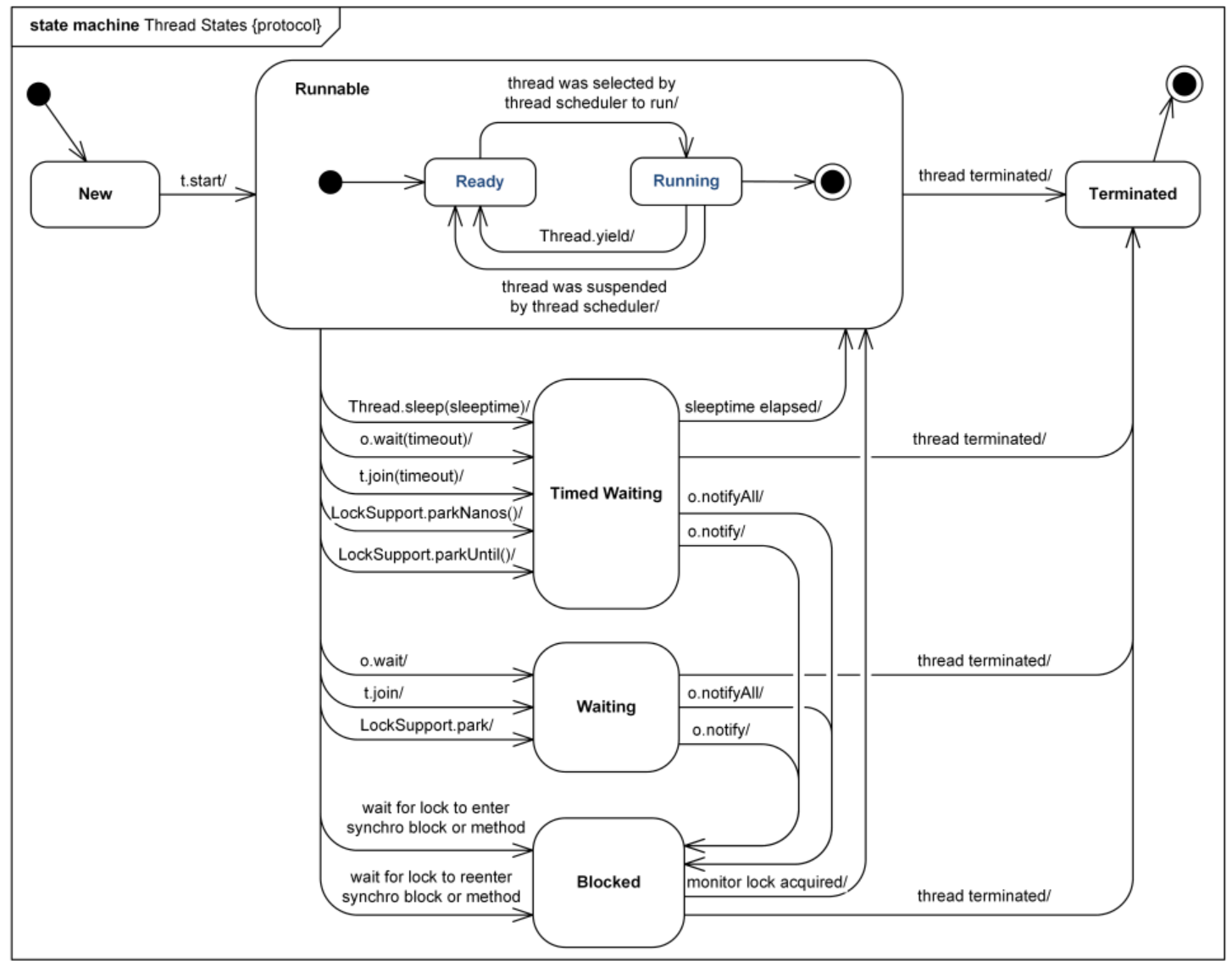
Image Source: Protocol state machine example - Thread states and life cycle in Java 6
Life Cycle of A Thread
- new: The thread is in new state if you create an instance of Thread class but before the invocation of start() method.
- runnable: The thread is in runnable state after invocation of start() method, but the thread scheduler has not selected it to be the running thread.
At the lower operating system (OS) level, JVM’s runnable state could be considered as a composite state with two substates. When a thread transitions to the runnable JVM state, the thread first goes into the ready substate. Thread scheduling decides when the thread could actually start, proceed or be suspended.Thread.yield()
is explicit recommendation to thread scheduler to pause the currently executing thread to allow some other thread to execute.
A thread in the runnable state is executing from the JVM point of view but in fact it may be waiting for some resources from the operating system. - time waiting: Timed waiting is a thread state for a thread waiting with a specified waiting time. A thread is in the timed waiting state due to calling one of the following methods with a specified positive waiting time:
Thread.sleep(sleeptime)
Thread.join(timeout)
LockSupport.partNanos(timeout)
LockSupport.partUntil(timeout)
- Not Runnable(Blocked/Waiting States): This is the state when the thread is still alive, but is currently not eligible to run.
1). blocking: Thread state for a thread blocked waiting for a monitor lock. A thread in the blocked state is waiting for a monitor lock to enter a synchronized block/method or reenter a synchronized block/method after calling.
2). waiting: A thread in the waiting state is waiting for another thread to perform a particular action. For example, a thread that has called Object.wait() on an object is waiting for another thread to call Object.notify() or Object.notifyAll() on that object. A thread is in the waiting state due to the calling one of the following methods without timeout:Thread.join()
LockSupport.park()
Note: if the thread is in the waiting states, it will not compete for the lock. After other threads calling Object.notify() or Object.notifyAll() on that object, then this thread goes to blocked states, ready to compete the lock.
5. Terminated: A thread is in terminated or dead state when its run() method exits.
Java API - Class Thread
1. public Thread.State getState()
Returns the state of this thread. This method is designed for use in monitoring of the system state, not for synchronization control.
2. java.lang.Thread.State class
Defines the ENUM constants for the state of a thread.
- Constant type: New
Declaration: public static final Thread.State NEW
- Constant type: Runnable
Declaration: public static final Thread.State RUNNABLE
- Constant type: Blocked
Declaration: public static final Thread.State BLOCKED
- Constant type: Waiting
Declaration: public static final Thread.State WAITING
- Constant type: Timed Waiting
Declaration: public static final Thread.State TIMED_WAITING
- Constant type: Terminated
Declaration: public static final Thread.State TERMINATED